Jenkinsfile#
Overview#
Note
This is a legacy content - and it may not be up-to-date.
Some time ago (long time ago) I was working with Jenkins - and I was using Jenkinsfile to define the pipeline.
Following examples are from that time - maybe they will be useful for someone (not sure if are still valid).
Example 01 - Run directly docker and docker-compose#
Tip
This is really nice example how Jenkins can work with
docker
anddocker-compose
.This is a simple example to use
goss
to test the docker container.
Code:
pipeline {
options {
disableConcurrentBuilds()
skipDefaultCheckout(true)
}
agent {
node {
label "custom-label"
}
}
post {
always {
script {
try {
sh "docker-compose rm -fsv"
} catch (e) {
echo "error in removing acceptance test containers"
}
}
deleteDir()
}
}
stages {
stage('Checkout SCM') {
steps {
echo 'Checking code in agent'
checkout scm
}
}
stage('Report / Check Environment') {
parallel {
stage('Details about builds') {
steps {
echo "Running ${env.BUILD_ID} on ${env.JENKINS_URL}"
}
}
stage('Dummy: Lint 01') {
steps {
echo 'Lint 01'
}
}
stage('Dummy: Lint 02') {
steps {
echo 'Lint 02'
}
}
}
}
stage("Run stack") {
steps {
echo "Starting stacks"
sh "docker-compose up -d"
sh "docker-compose ps"
}
}
stage("Test containers with goss") {
parallel {
stage('Testing on nginx01-test01') {
steps {
echo "Check goss:"
sh "docker exec nginx01-test01 ls -la /goss_tests/"
sh "docker exec nginx01-test01 /goss/goss -g /goss_tests/nginx_default.yaml validate --color --format tap"
}
}
stage('Testing on nginx02-test01') {
steps {
echo "Check goss:"
sh "docker exec nginx02-test01 ls -la /goss"
sh "docker exec nginx02-test01 /goss/goss -g /goss_tests/nginx_default.yaml validate --color --format tap"
}
}
}
}
}
}
And this is a result:
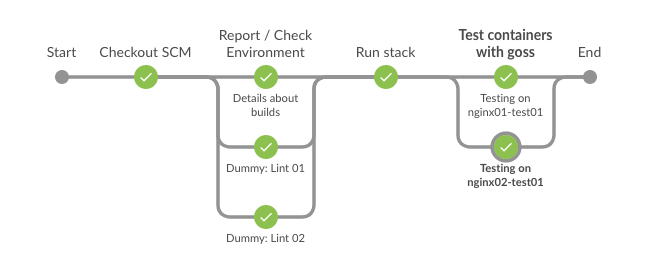
Example 02 - Run docker via Jenkins plugin#
Following example will run ansible from docker container - and will use credentails from Jenkins.
Code:
#!groovy
pipeline {
agent {
node {
label "custom-label"
}
}
// cleanup and notifications (for each agent)
post {
always {
deleteDir()
}
}
options {
disableConcurrentBuilds()
}
stages {
// Echo Environment
stage ("init") {
steps {
sh "pwd"
sh "bash --version"
sh "env"
}
}
// https://jenkins.io/doc/book/pipeline/docker/
stage ("Main") {
agent {
docker {
reuseNode true
// !!! IMPORTANT: Not sure why hardcoded name must be used and not variable !!!
// https://stackoverflow.com/questions/53882492/jenkins-how-to-use-variables-inside-agent-docker-args
image 'registry/ansible:0.1.0'
}
}
steps {
// Utilise the Jenkins SSH plugin to call into the host where we know Ansible is running. In this case
sshagent(['832bd9f4840-4f4e-6a81-8981-aeba9e090']) {
script {
sh "ansible/call-ansible.sh"
}
}
}
}
}
}